Exploring the Broadcast Channel API for cross-tab communication
Discover how the Broadcast Channel API enables seamless cross-tab communication in web applications. This API allows different browser tabs to exchange messages efficiently, improving real-time data synchronization and user experience across multiple open tabs within the same application.
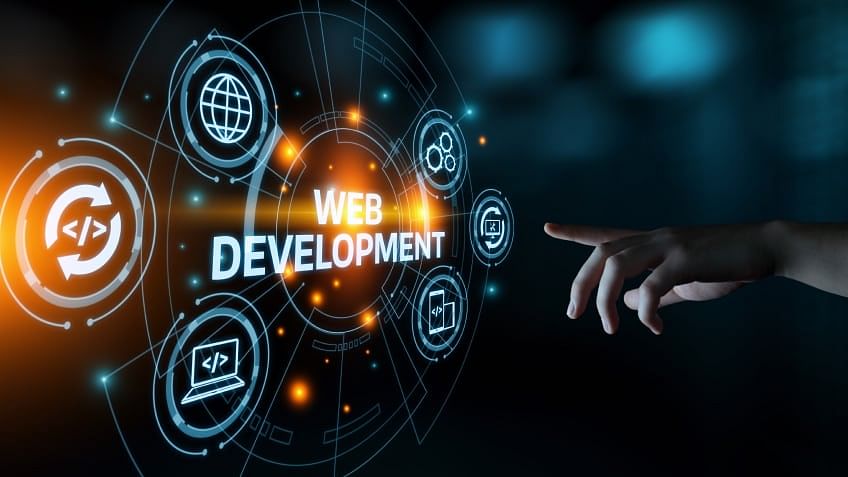
Understanding the Broadcast Channel API
The Broadcast Channel API is a powerful tool that enables communication between different browsing contexts, such as multiple tabs, windows, or even iframes within the same origin. This API provides a way for scripts in separate contexts to send messages to each other, effectively creating a communication channel that facilitates seamless data sharing across different parts of a web application. Whether you're developing a single-page application (SPA) or a more complex web application, the Broadcast Channel API offers a robust solution for synchronizing state and data across various tabs and windows.
The Importance of Cross-Tab Communication
In modern web applications, user experience and functionality are paramount. Users often interact with web applications in multiple tabs or windows, and ensuring that the application remains consistent across all these contexts is essential. Cross-tab communication allows developers to synchronize user actions, preferences, and data in real time, providing a seamless and cohesive experience. The Broadcast Channel API simplifies this process, enabling developers to build more responsive and user-friendly applications.
How the Broadcast Channel API Works
The Broadcast Channel API operates by creating a named communication channel that multiple contexts can join. Once a context joins the channel, it can send and receive messages from other contexts in the same channel. This process is asynchronous, meaning that messages are sent and received without blocking the main thread, ensuring smooth performance.
When a context wants to send a message, it broadcasts the message to the channel. All contexts that have joined the channel and are listening for messages will receive the broadcasted message. This allows for efficient communication between different parts of an application, such as synchronizing data, updating user interfaces, or handling user actions.
Use Cases for the Broadcast Channel API
The Broadcast Channel API has a wide range of applications in modern web development. Some of the most common use cases include:
Session Management: Synchronizing user sessions across multiple tabs to ensure that users remain logged in and their session data is consistent across different contexts.
Real-Time Collaboration: Enabling real-time collaboration features, such as document editing or chat applications, where changes made in one tab are instantly reflected in other open tabs.
Notifications: Sending notifications to other tabs when certain events occur, such as receiving a new message or updating user data.
State Synchronization: Keeping the application state consistent across multiple tabs, ensuring that changes made in one tab are reflected in others.
Broadcasting User Actions: Sharing user actions, such as clicking a button or submitting a form, across multiple tabs to provide a synchronized experience.
Implementing the Broadcast Channel API
Implementing the Broadcast Channel API is straightforward. The first step is to create a new BroadcastChannel object, specifying the name of the channel. Once the channel is created, you can start sending and receiving messages.
Here's a basic example of how to use the Broadcast Channel API:
const channel = new BroadcastChannel('my_channel');
// Sending a message
channel.postMessage('Hello from tab 1');
// Receiving a message
channel.onmessage = (event) => {
console.log('Received message:', event.data);
};
// Creating a new Broadcast Channel const channel = new BroadcastChannel('my_channel'); // Sending a message channel.postMessage('Hello from tab 1'); // Receiving a message channel.onmessage = (event) => { console.log('Received message:', event.data); };
In this example, a new BroadcastChannel object is created with the name 'my_channel'. The postMessage
method is used to send a message to the channel, and the onmessage
event handler is used to receive and handle messages.
Handling Different Types of Messages
The Broadcast Channel API allows you to send and receive different types of messages, including strings, objects, and more. This flexibility enables developers to pass complex data structures between different contexts, making it easier to synchronize state and data.
For example, you can send an object containing user data from one tab to another:
channel.postMessage({ user: 'John Doe', status: 'active' });
// Receiving the object message
channel.onmessage = (event) => {
console.log('Received user data:', event.data);
};
// Sending an object as a message channel.postMessage({ user: 'John Doe', status: 'active' }); // Receiving the object message channel.onmessage = (event) => { console.log('Received user data:', event.data); };
This example demonstrates how you can send an object with user data through the Broadcast Channel API and handle the received data in another context.
Managing Multiple Channels
In some cases, you might need to manage multiple channels within your application. The Broadcast Channel API supports creating and managing multiple channels, each with its own unique name. This allows you to organize your communication logic and separate different types of messages into different channels.
For instance, you might create separate channels for different parts of your application:
const userChannel = new BroadcastChannel('user_channel');
const notificationChannel = new BroadcastChannel('notification_channel');
// Sending messages on different channels
userChannel.postMessage({ user: 'John Doe', status: 'active' });
notificationChannel.postMessage('New notification received');
// Receiving messages on different channels
userChannel.onmessage = (event) => {
console.log('User data received:', event.data);
};
notificationChannel.onmessage = (event) => {
console.log('Notification received:', event.data);
};
// Creating multiple channels const userChannel = new BroadcastChannel('user_channel'); const notificationChannel = new BroadcastChannel('notification_channel'); // Sending messages on different channels userChannel.postMessage({ user: 'John Doe', status: 'active' }); notificationChannel.postMessage('New notification received'); // Receiving messages on different channels userChannel.onmessage = (event) => { console.log('User data received:', event.data); }; notificationChannel.onmessage = (event) => { console.log('Notification received:', event.data); };
This approach allows you to keep your communication logic organized and ensures that different types of messages are handled appropriately.
Best Practices for Using the Broadcast Channel API
To make the most of the Broadcast Channel API, it's essential to follow best practices that ensure efficient and reliable communication. Some of these best practices include:
Channel Naming Conventions: Use clear and descriptive names for your channels to avoid confusion and ensure that messages are sent and received on the correct channels.
Message Validation: Always validate and sanitize incoming messages to prevent potential security issues and ensure that your application handles messages correctly.
Error Handling: Implement error handling to catch and respond to any issues that may arise during communication, such as network errors or unexpected message formats.
Performance Considerations: Be mindful of the performance impact of sending and receiving messages, especially in applications with high traffic or complex data structures. Optimize your code to minimize any potential performance bottlenecks.
Security: While the Broadcast Channel API is limited to communication within the same origin, it's still important to consider security implications and ensure that your application does not inadvertently expose sensitive data.
The Future of Cross-Tab Communication
The Broadcast Channel API represents a significant step forward in cross-tab communication, offering a simple and effective way to synchronize data and state across different contexts. As web applications continue to evolve, the need for robust cross-tab communication will only grow, making the Broadcast Channel API an increasingly valuable tool for developers.
Looking ahead, we can expect further improvements and enhancements to the API, potentially expanding its capabilities and making it even more powerful. As more developers adopt the Broadcast Channel API, best practices and patterns will emerge, helping to standardize and optimize its use in modern web development.
Leveraging the Broadcast Channel API for Business Success
For businesses, the Broadcast Channel API offers numerous opportunities to enhance web applications and provide a better user experience. By leveraging this API, companies can create more responsive and cohesive applications, leading to increased user satisfaction and engagement.
Whether you're building an e-commerce platform, a content management system, or a real-time collaboration tool, the Broadcast Channel API can help you achieve your business goals by enabling efficient communication between different parts of your application. This, in turn, can lead to higher conversion rates, improved customer retention, and ultimately, greater business success.
Integrating the Broadcast Channel API into Your Development Workflow
Integrating the Broadcast Channel API into your development workflow is a straightforward process that can yield significant benefits. Start by identifying the parts of your application that would benefit from cross-tab communication, such as user sessions, notifications, or real-time collaboration features.
Once you've identified the use cases, you can begin implementing the Broadcast Channel API in your application. Follow the best practices outlined above to ensure that your implementation is efficient, secure, and reliable.
As you integrate the API, it's essential to test your application thoroughly to ensure that communication between different contexts is working as expected. This includes testing in various scenarios, such as multiple tabs, windows, and devices, to ensure that your application provides a consistent experience for all users.
Exploring Advanced Features and Use Cases
While the basic functionality of the Broadcast Channel API is powerful on its own, there are advanced features and use cases that can further enhance your application. For example, you can use the API in conjunction with other web technologies, such as Service Workers or WebSockets, to create even more dynamic and responsive applications.
Additionally, you can explore use cases beyond the traditional web application context, such as integrating the Broadcast Channel API into progressive web apps (PWAs) or using it in combination with other APIs to create unique and innovative user experiences.
As you explore these advanced features and use cases, you'll discover new ways to leverage the Broadcast Channel API to create cutting-edge web applications that stand out in a competitive market.
FAQ
What is the Broadcast Channel API?
The Broadcast Channel API is a web API that allows communication between different browsing contexts (such as tabs, windows, or iframes) within the same origin, enabling data sharing and synchronization across these contexts.
How does the Broadcast Channel API work?
The API creates a named communication channel that multiple contexts can join. Once joined, contexts can send and receive messages through this channel, enabling cross-tab communication.
What are the benefits of using the Broadcast Channel API?
The API allows for real-time synchronization of data and state across multiple tabs or windows, providing a seamless user experience. It's particularly useful for session management, real-time collaboration, notifications, and state synchronization.
Can I send different types of messages through the Broadcast Channel API?
Yes, the Broadcast Channel API supports sending various types of messages, including strings, objects, and more, making it flexible for different communication needs.
Is the Broadcast Channel API secure?
While the Broadcast Channel API is restricted to communication within the same origin, it's essential to validate and sanitize incoming messages to prevent potential security risks.
How can businesses benefit from the Broadcast Channel API?
Businesses can use the API to enhance
Get in Touch
Website – https://www.webinfomatrix.com
Mobile - +91 9212306116
Whatsapp – https://call.whatsapp.com/voice/9rqVJyqSNMhpdFkKPZGYKj
Skype – shalabh.mishra
Telegram – shalabhmishra
Email - info@webinfomatrix.com
What's Your Reaction?

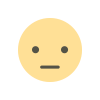
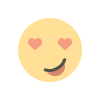

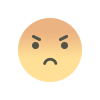
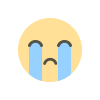
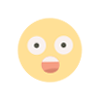